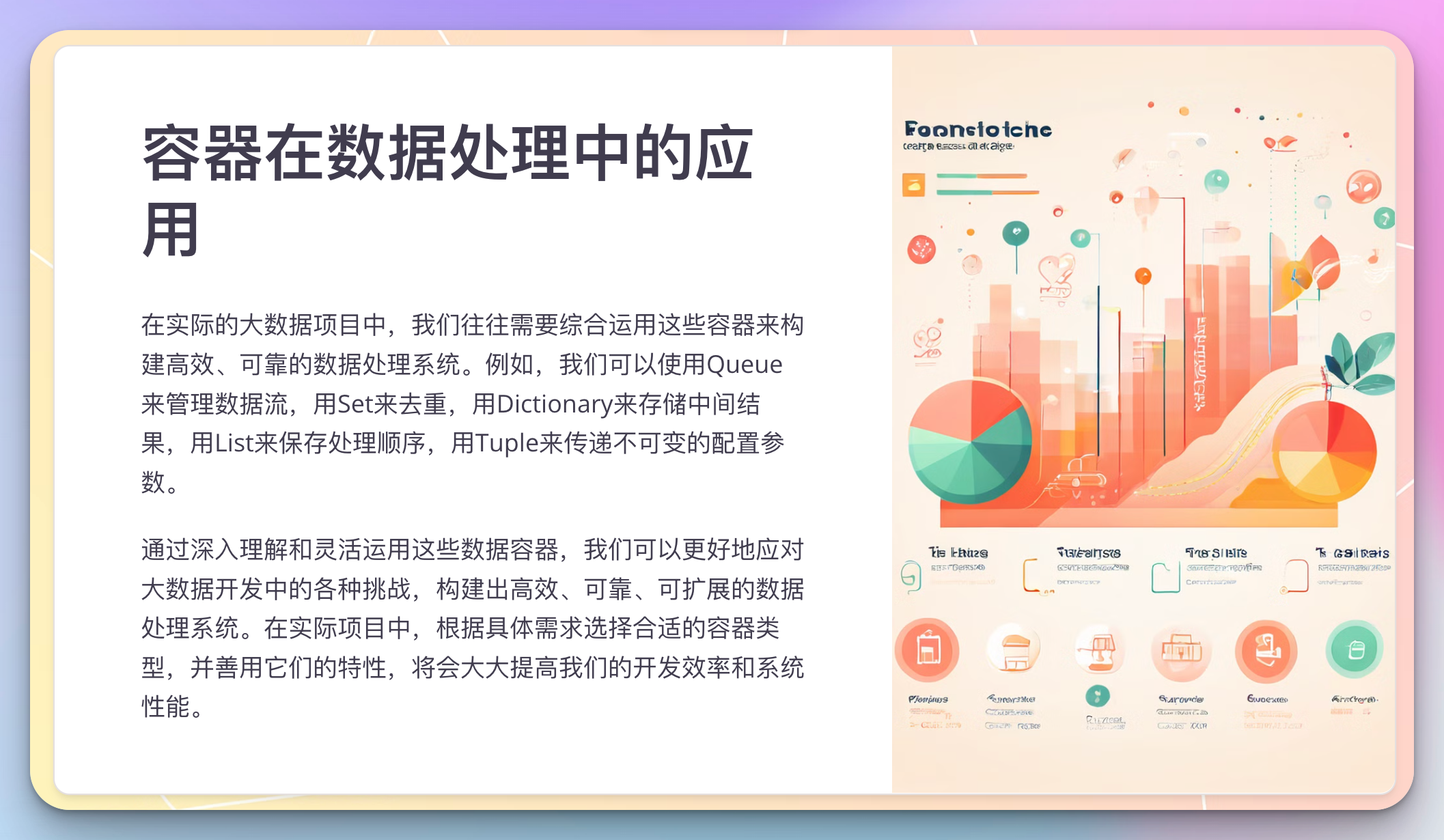
Python中的数据容器及其在大数据开发中的应用
在大数据开发中,选择合适的数据容器不仅可以提高代码的效率,还能增强系统的可靠性和可维护性。Set适用于需要去重和快速成员检测的场景。List适合保持元素顺序和支持随机访问的情况。Dictionary在需要快速查找和复杂数据结构时非常有用。Tuple在需要不可变序列的场景下发挥作用,如多线程中的共享数据。Queue在并发编程和数据流处理中尤其有用,能实现高效的生产者-消费者模型。在实际的大数据项目中
在Python编程中,数据容器是存储和组织数据的基本工具。作为大数据开发者,了解并灵活运用各种容器类型对于高效处理大规模数据至关重要。今天,我们将从Set出发,探讨Python中的各种数据容器,以及它们在大数据处理中的应用。
目录
1. Set:独特元素的容器
Set是Python中一个非常特殊的容器类型,它只存储唯一的元素。
unique_visitors = {'user1', 'user2', 'user3', 'user1'}
print(unique_visitors) # 输出: {'user1', 'user2', 'user3'}
1.1 去重的力量
故事1: 在一个大型电商平台的日志分析项目中,我们需要计算每日的独立访客数。使用Set可以轻松去除重复的用户ID:
daily_logs = ['user1', 'user2', 'user1', 'user3', 'user2', 'user4']
unique_daily_visitors = set(daily_logs)
print(f"独立访客数:{len(unique_daily_visitors)}")
故事2: 在基因研究中,科学家们经常需要找出DNA序列中的唯一片段。Set可以快速实现这一目标:
dna_fragments = ['ATCG', 'GCTA', 'ATCG', 'TGCA', 'GCTA']
unique_fragments = set(dna_fragments)
print(f"唯一的DNA片段:{unique_fragments}")
故事3: 在社交网络分析中,我们可能需要找出两个用户的共同好友。使用Set的交集操作可以轻松实现:
user1_friends = {'Alice', 'Bob', 'Charlie', 'David'}
user2_friends = {'Bob', 'Charlie', 'Eve', 'Frank'}
common_friends = user1_friends & user2_friends
print(f"共同好友:{common_friends}")
2. List:有序元素的容器
与Set不同,List是一个有序的容器,允许重复元素。
user_actions = ['click', 'scroll', 'click', 'purchase']
print(user_actions) # 输出: ['click', 'scroll', 'click', 'purchase']
2.1 保持顺序的重要性
故事1: 在一个用户行为分析项目中,我们需要追踪用户的操作序列:
def analyze_user_journey(actions):
if actions[-1] == 'purchase':
return "转化成功"
elif 'add_to_cart' in actions:
return "潜在客户"
else:
return "需要更多互动"
user1_journey = ['view', 'click', 'add_to_cart', 'purchase']
print(analyze_user_journey(user1_journey)) # 输出: 转化成功
故事2: 在自然语言处理中,单词的顺序对于理解句子含义至关重要:
def simple_sentiment_analysis(words):
positive = ['good', 'great', 'excellent']
negative = ['bad', 'terrible', 'awful']
score = sum(1 if word in positive else -1 if word in negative else 0 for word in words)
return "正面" if score > 0 else "负面" if score < 0 else "中性"
sentence = ['the', 'product', 'is', 'not', 'bad']
print(simple_sentiment_analysis(sentence)) # 输出: 负面
故事3: 在时间序列分析中,数据的顺序代表了时间的流逝:
import statistics
def detect_anomaly(time_series, window_size=3, threshold=2):
anomalies = []
for i in range(len(time_series) - window_size + 1):
window = time_series[i:i+window_size]
mean = statistics.mean(window)
std = statistics.stdev(window)
if abs(window[-1] - mean) > threshold * std:
anomalies.append(i + window_size - 1)
return anomalies
data = [1, 2, 3, 2, 100, 3, 4]
print(f"异常点索引:{detect_anomaly(data)}") # 输出: 异常点索引:[4]
3. Dictionary:键值对的容器
Dictionary是Python中最灵活的容器之一,它存储键值对,允许通过键快速访问值。
user_info = {'name': 'Alice', 'age': 30, 'occupation': 'Data Scientist'}
print(user_info['occupation']) # 输出: Data Scientist
3.1 高效的数据映射
故事1: 在处理大规模日志数据时,我们可能需要快速统计各种事件的发生次数:
from collections import defaultdict
def count_events(logs):
event_counts = defaultdict(int)
for event in logs:
event_counts[event] += 1
return dict(event_counts)
logs = ['login', 'view_page', 'click', 'login', 'purchase', 'view_page']
print(count_events(logs))
故事2: 在构建推荐系统时,我们可能需要维护用户的偏好数据:
user_preferences = {
'user1': {'sci-fi': 0.8, 'action': 0.6, 'romance': 0.2},
'user2': {'comedy': 0.7, 'drama': 0.5, 'sci-fi': 0.3}
}
def recommend_genre(user, preferences):
if user in preferences:
return max(preferences[user], key=preferences[user].get)
return "No recommendation available"
print(recommend_genre('user1', user_preferences)) # 输出: sci-fi
故事3: 在网络分析中,我们可能需要使用字典来表示图结构:
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
def find_all_paths(graph, start, end, path=[]):
path = path + [start]
if start == end:
return [path]
if start not in graph:
return []
paths = []
for node in graph[start]:
if node not in path:
newpaths = find_all_paths(graph, node, end, path)
for newpath in newpaths:
paths.append(newpath)
return paths
print(find_all_paths(graph, 'A', 'F'))
非常好,让我们继续深入探讨Python中的数据容器及其在大数据开发中的应用。
小结
在大数据开发中,选择合适的数据容器对于实现高效的数据处理至关重要。
Set适用于需要去重和集合运算的场景,List适合保持元素顺序很重要的情况,而Dictionary则在需要快速查找和复杂数据结构时非常有用。
通过灵活运用这些容器,我们可以更好地组织和处理大规模数据。例如,在处理用户行为数据时,我们可能会使用Set来找出独特用户,使用List来保存用户的行为序列,使用Dictionary来存储用户的详细信息和偏好。
# 综合示例
user_data = {
'unique_users': set(),
'user_journeys': defaultdict(list),
'user_profiles': {}
}
def process_user_action(user_id, action):
user_data['unique_users'].add(user_id)
user_data['user_journeys'][user_id].append(action)
if user_id not in user_data['user_profiles']:
user_data['user_profiles'][user_id] = {'actions_count': 0}
user_data['user_profiles'][user_id]['actions_count'] += 1
# 模拟数据处理
process_user_action('user1', 'login')
process_user_action('user2', 'view_page')
process_user_action('user1', 'purchase')
print(f"独立用户数: {len(user_data['unique_users'])}")
print(f"用户1的行为序列: {user_data['user_journeys']['user1']}")
print(f"用户1的概况: {user_data['user_profiles']['user1']}")
通过深入理解和灵活运用这些数据容器,我们可以构建更高效、更强大的大数据处理系统。
在实际项目中,往往需要结合使用多种容器类型来解决复杂的数据处理问题。
4. Tuple:不可变序列容器
Tuple是Python中的一种不可变序列,一旦创建就不能修改。这种特性使得Tuple在某些场景下特别有用。
coordinates = (40.7128, -74.0060) # 纽约市的经纬度
print(coordinates[0]) # 输出: 40.7128
4.1 不可变性的优势
故事1: 在地理信息系统(GIS)中,我们经常需要处理大量的坐标数据。使用Tuple可以确保坐标不被意外修改:
def calculate_distance(point1, point2):
from math import radians, sin, cos, sqrt, atan2
lat1, lon1 = map(radians, point1)
lat2, lon2 = map(radians, point2)
dlat = lat2 - lat1
dlon = lon2 - lon1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * atan2(sqrt(a), sqrt(1-a))
R = 6371 # 地球半径(公里)
return R * c
new_york = (40.7128, -74.0060)
los_angeles = (34.0522, -118.2437)
distance = calculate_distance(new_york, los_angeles)
print(f"纽约到洛杉矶的距离约为 {distance:.2f} 公里")
故事2: 在数据库操作中,使用Tuple可以安全地传递多个参数:
import sqlite3
def insert_user(conn, user_data):
cursor = conn.cursor()
cursor.execute("INSERT INTO users (name, age, email) VALUES (?, ?, ?)", user_data)
conn.commit()
conn = sqlite3.connect(':memory:')
conn.execute("CREATE TABLE users (name TEXT, age INTEGER, email TEXT)")
new_user = ('Alice', 30, 'alice@example.com')
insert_user(conn, new_user)
# 验证插入
cursor = conn.execute("SELECT * FROM users")
print(cursor.fetchone()) # 输出: ('Alice', 30, 'alice@example.com')
故事3: 在多线程编程中,Tuple可以用作不可变的共享数据结构:
import threading
shared_data = (10, 20, 30) # 不可变的共享数据
def worker(data):
print(f"线程 {threading.current_thread().name} 读取数据: {data}")
# 尝试修改数据会引发错误
# data[0] = 100 # 这行会引发 TypeError
threads = []
for i in range(3):
t = threading.Thread(target=worker, args=(shared_data,))
threads.append(t)
t.start()
for t in threads:
t.join()
5. Queue:先进先出的容器
Queue是一种特殊的容器,遵循先进先出(FIFO)原则,在并发编程和数据流处理中非常有用。
from queue import Queue
task_queue = Queue()
task_queue.put("Task 1")
task_queue.put("Task 2")
print(task_queue.get()) # 输出: Task 1
5.1 队列在数据处理中的应用
故事1: 在日志处理系统中,使用Queue可以实现高效的生产者-消费者模型:
import threading
import time
from queue import Queue
log_queue = Queue()
def log_producer():
for i in range(5):
log_entry = f"Log entry {i}"
log_queue.put(log_entry)
print(f"Produced: {log_entry}")
time.sleep(0.5)
def log_consumer():
while True:
log_entry = log_queue.get()
if log_entry is None:
break
print(f"Consumed: {log_entry}")
log_queue.task_done()
# 启动生产者线程
producer_thread = threading.Thread(target=log_producer)
producer_thread.start()
# 启动消费者线程
consumer_thread = threading.Thread(target=log_consumer)
consumer_thread.start()
# 等待生产者完成
producer_thread.join()
# 发送终止信号给消费者
log_queue.put(None)
# 等待消费者完成
consumer_thread.join()
故事2: 在实时数据处理流水线中,Queue可以用来连接不同的处理阶段:
import threading
from queue import Queue
data_queue = Queue()
processed_queue = Queue()
def data_generator():
for i in range(10):
data = f"Data {i}"
data_queue.put(data)
data_queue.put(None) # 发送结束信号
def data_processor():
while True:
data = data_queue.get()
if data is None:
processed_queue.put(None)
break
processed_data = f"Processed {data}"
processed_queue.put(processed_data)
def data_writer():
while True:
data = processed_queue.get()
if data is None:
break
print(f"Writing: {data}")
# 启动线程
threading.Thread(target=data_generator).start()
threading.Thread(target=data_processor).start()
threading.Thread(target=data_writer).start()
故事3: 在大规模Web爬虫系统中,Queue可以用来管理待爬取的URL:
import threading
from queue import Queue
import time
import random
url_queue = Queue()
results = []
def url_producer():
for i in range(20):
url = f"http://example.com/page{i}"
url_queue.put(url)
# 添加结束标记
for _ in range(3): # 假设有3个消费者线程
url_queue.put(None)
def url_consumer():
while True:
url = url_queue.get()
if url is None:
break
# 模拟爬取过程
time.sleep(random.uniform(0.1, 0.5))
results.append(f"Crawled: {url}")
url_queue.task_done()
# 启动生产者线程
producer = threading.Thread(target=url_producer)
producer.start()
# 启动消费者线程
consumers = []
for _ in range(3):
consumer = threading.Thread(target=url_consumer)
consumers.append(consumer)
consumer.start()
# 等待所有线程完成
producer.join()
for consumer in consumers:
consumer.join()
print(f"爬取完成,总共爬取了 {len(results)} 个页面")
总结
在大数据开发中,选择合适的数据容器不仅可以提高代码的效率,还能增强系统的可靠性和可维护性。我们探讨了Set、List、Dictionary、Tuple和Queue这几种常用的数据容器,每种容器都有其独特的特性和适用场景:
- Set适用于需要去重和快速成员检测的场景。
- List适合保持元素顺序和支持随机访问的情况。
- Dictionary在需要快速查找和复杂数据结构时非常有用。
- Tuple在需要不可变序列的场景下发挥作用,如多线程中的共享数据。
- Queue在并发编程和数据流处理中尤其有用,能实现高效的生产者-消费者模型。
在实际的大数据项目中,我们往往需要综合运用这些容器来构建高效、可靠的数据处理系统。例如,我们可以使用Queue来管理数据流,用Set来去重,用Dictionary来存储中间结果,用List来保存处理顺序,用Tuple来传递不可变的配置参数。
import threading
from queue import Queue
from collections import defaultdict
class DataProcessor:
def __init__(self):
self.input_queue = Queue()
self.output_queue = Queue()
self.unique_items = set()
self.item_counts = defaultdict(int)
self.processing_order = []
self.config = ('config1', 'config2', 'config3')
def process_data(self):
while True:
item = self.input_queue.get()
if item is None:
break
# 使用Set去重
if item not in self.unique_items:
self.unique_items.add(item)
# 使用Dictionary计数
self.item_counts[item] += 1
# 使用List记录处理顺序
self.processing_order.append(item)
# 使用Tuple读取不可变配置
processed_item = f"Processed {item} with {self.config}"
self.output_queue.put(processed_item)
self.input_queue.task_done()
def run(self):
# 启动处理线程
processor_thread = threading.Thread(target=self.process_data)
processor_thread.start()
# 模拟数据输入
for i in range(20):
self.input_queue.put(f"Item{i%5}")
self.input_queue.put(None) # 发送结束信号
# 等待处理完成
processor_thread.join()
# 输出结果
print(f"唯一项目数: {len(self.unique_items)}")
print(f"项目计数: {dict(self.item_counts)}")
print(f"处理顺序: {self.processing_order}")
print("处理后的项目:")
while not self.output_queue.empty():
print(self.output_queue.get())
# 运行数据处理器
processor = DataProcessor()
processor.run()
通过深入理解和灵活运用这些数据容器,我们可以更好地应对大数据开发中的各种挑战,构建出高效、可靠、可扩展的数据处理系统。在实际项目中,根据具体需求选择合适的容器类型,并善用它们的特性,将会大大提高我们的开发效率和系统性能。
更多推荐
所有评论(0)