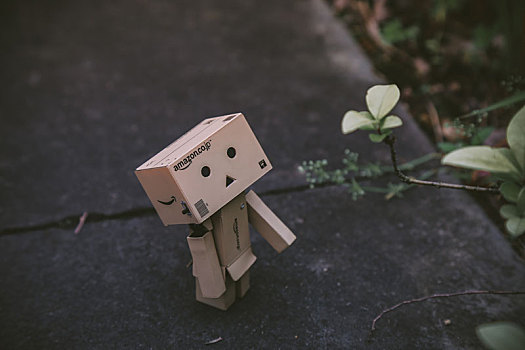
鸿蒙HarmonyOS NEXT开发实战:HTTP发起网络数据请求NAPI封装(C/C++)
发送一个同步HTTP请求,也可以设置请求头和请求体等参数,并返回来自服务器的HTTP响应。常用于获取资源,支持通过拦截器来处理请求和响应。
鸿蒙开发实战往期文章必看:
一分钟了解”纯血版!鸿蒙HarmonyOS Next应用开发!
“非常详细的” 鸿蒙HarmonyOS Next应用开发学习路线!(从零基础入门到精通)
“一杯冰美式的时间” 了解鸿蒙HarmonyOS Next应用开发路径!
使用fetchsync发送同步网络请求 (C/C++)
场景介绍
发送一个同步HTTP请求,也可以设置请求头和请求体等参数,并返回来自服务器的HTTP响应。常用于获取资源,支持通过拦截器来处理请求和响应。
接口说明
具体API说明详见接口文档。
接口名 | 描述 |
---|---|
Rcp_Response *HMS_Rcp_FetchSync(Rcp_Session *session, Rcp_Request *request, uint32_t *errCode); | 发送一个HTTP请求,并直接返回来自服务器的HTTP响应。 |
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建Request对象。"https://www.example.com"请根据实际情况替换为想要请求的URL地址。(实际使用时请将该代码块放入main函数或者其他函数区域内)。
const char *kHttpServerAddress = "http://www.example.com"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress);
- 创建会话。(实际使用时请将该代码块放入main函数或者其他函数区域内)。
uint32_t errCode = 0; Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode);
- 发起请求,并处理返回结果。(实际使用时请将该代码块放入main函数或者其他函数区域内)。
Rcp_Response *response = HMS_Rcp_FetchSync(session, request, &errCode); if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); }
- 清理response响应和request请求。最后关闭session。(实际使用时请将该代码块放入main函数或者其他函数区域内)。
// 清理request HMS_Rcp_DestroyRequest(request); // 处理response,并清理response if (response != NULL) { response->destroyResponse(response); } // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode
使用fetch发起异步网络请求 (C/C++)
场景介绍
发送一个异步HTTP请求,也可以设置请求头和请求体等参数,并返回来自服务器的HTTP响应。常用于获取资源,支持通过拦截器来处理请求和响应。
接口说明
具体API说明详见接口文档。
接口名 | 描述 |
---|---|
uint32_t HMS_Rcp_Fetch(Rcp_Session *session, Rcp_Request *request, const Rcp_ResponseCallbackObject *responseCallback); | 发送一个HTTP请求,并返回来自服务器的HTTP响应。使用responseCallback异步回调。 |
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建Request对象。"https://www.example.com"请根据实际情况替换为想要请求的URL地址。(完整见步骤5)
const char *kHttpServerAddress = "http://www.example.com"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress);
- 创建会话。(完整见步骤5)
uint32_t errCode = 0; Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode);
- 发起请求,并处理返回结果。最后关闭session。
bool g_callback = false; // 异步请求的响应处理回调,请用户自定义 void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } // 注意清理响应 if (response != NULL) { response->destroyResponse(response); } g_callback = true; } int main() { const char *kHttpServerAddress = "http://www.example.com"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_GET; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 处理errCode // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode return 0; }
使用get发送网络请求 (C/C++)
场景介绍
发送一个带有默认HTTP参数的HTTP GET请求,并返回来自服务器的HTTP响应。使用异步回调。常用于从服务器获取数据。
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起get请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } // 注意清理响应 if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_GET; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 处理errCode // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode return 0; }
使用post发送网络请求 (C/C++)
场景介绍
发送一个带有默认HTTP参数的HTTP POST请求,并返回来自服务器的HTTP响应。使用异步回调。常用于向服务器提交数据。与GET请求不同,POST请求将参数包含在请求主体中,适用于创建新资源、提交表单数据或执行某些操作。
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <cstdlib> #include <cstring> #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起post请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } // 注意清理响应 if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com"; const char *content = "content"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); // 设置request参数 request->method = RCP_METHOD_POST; request->content = (Rcp_RequestContent *)calloc(1, sizeof(Rcp_RequestContent)); request->content->type = RCP_CONTENT_TYPE_STRING; request->content->data.contentStr.buffer = content; request->content->data.contentStr.length = strlen(content); uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 处理errCode // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 清理request HMS_Rcp_DestroyRequest(request); // 清理request->content free(request->content); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode return 0; }
使用put发送网络请求 (C/C++)
场景介绍
发送一个带有默认HTTP参数的HTTP PUT请求,并返回来自服务器的HTTP响应。使用异步回调。常用于向服务器更新资源。PUT请求将更新的数据发送到特定的URL,用于替换指定资源的全部内容。
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <cstdlib> #include <cstring> #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起put请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } // 注意清理响应 if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com"; const char *content = "content"; // 创建request,并设置request的参数 Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_PUT; request->content = (Rcp_RequestContent *)calloc(1, sizeof(Rcp_RequestContent)); request->content->type = RCP_CONTENT_TYPE_STRING; request->content->data.contentStr.buffer = content; request->content->data.contentStr.length = strlen(content); uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起fetch请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 等待结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode // 清理request content free(request->content); return 0; }
使用head发送网络请求 (C/C++)
场景介绍
发送一个带有默认HTTP参数的HTTP HEAD请求,并返回来自服务器的HTTP响应。使用异步回调。类似GET请求,但只返回相应头,不返回实体内容。可以获取资源的元信息,如文件大小、修改日期等。
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起head请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } // 注意清理响应 if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com/head"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_HEAD; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起fetch请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode }
使用delete发送网络请求 (C/C++)
场景介绍
发送一个带有默认HTTP参数的HTTP DELETE请求,并返回来自服务器的HTTP响应。使用异步回调。用于从服务器删除资源。通过向指定URL发送DELETE请求,可以删除该URL上对应的资源。
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起delete请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com/delete"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_DELETE; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起fetch请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode return 0; }
使用cancel取消网络请求 (C/C++)
场景介绍
取消指定或正在进行的会话请求。
接口说明
具体API说明详见接口文档。
接口名 | 描述 |
---|---|
uint32_t HMS_Rcp_CancelRequest(Rcp_Session *session, const Rcp_Request *request); | 取消指定或所有正在进行的会话请求。返回为空。 |
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起请求。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。在使用fetch请求后,使用HMS_Rcp_CancelRequest取消网路请求。销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com/delete"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_DELETE; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起fetch请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 取消请求,处理errCode errCode = HMS_Rcp_CancelRequest(session, request); // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 清理request HMS_Rcp_DestroyRequest(request); // 关闭session errCode = HMS_Rcp_CloseSession(&session); // 处理errCode return 0; }
使用close关闭会话 (C/C++)
场景介绍
远场通信请求结束后,需要关闭会话。调用此方法以释放与此会话关联的资源。
接口说明
具体API说明详见接口文档。
接口名 | 描述 |
---|---|
uint32_t HMS_Rcp_CloseSession(Rcp_Session **session); | 关闭会话。 |
使用示例
- CPP侧导入模块。
#include "RemoteCommunicationKit/rcp.h" #include <stdio.h> #include <unistd.h>
- CMakeLists.txt中添加以下lib。(具体请见C API开发准备)。
librcp_c.so
- 创建会话,会话发起请求后关闭会话。“http://www.example.com”请根据实际情况替换为想要请求的URL地址。等待响应返回后,销毁request并关闭session。
void ResponseCallback(void *usrCtx, Rcp_Response *response, uint32_t errCode) { (void *)usrCtx; if (response != NULL) { printf("Response status: %d\n", response->statusCode); } else { printf("Fetch failed: errCode: %u\n", errCode); } if (response != NULL) { response->destroyResponse(response); } } bool g_callback = false; int main() { const char *kHttpServerAddress = "http://www.example.com"; Rcp_Request *request = HMS_Rcp_CreateRequest(kHttpServerAddress); request->method = RCP_METHOD_GET; uint32_t errCode = 0; // 创建session Rcp_Session *session = HMS_Rcp_CreateSession(NULL, &errCode); // 配置请求回调 Rcp_ResponseCallbackObject responseCallback = {ResponseCallback, NULL}; // 发起fetch请求 errCode = HMS_Rcp_Fetch(session, request, &responseCallback); // 等待fetch结果 int timeout = 100; while (timeout-- > 0 && !g_callback) { usleep(1000); } // 在退出前取消可能还在执行的requests errCode = HMS_Rcp_CancelSession(session); // 处理errCode errCode = HMS_Rcp_CloseSession(&session); // 处理errCode HMS_Rcp_DestroyRequest(request); return 0; }
最后
小编在之前的鸿蒙系统扫盲中,我明显感觉到一点,那就是许多人参与鸿蒙开发,但是又不知道从哪里下手,有很多小伙伴不知道学习哪些鸿蒙开发技术?不知道需要重点掌握哪些鸿蒙应用开发知识点?而且学习时频繁踩坑,最终浪费大量时间。所以有一份实用的鸿蒙(HarmonyOS NEXT)路线图、文档、视频、用来跟着学习是非常有必要的。
如果你是一名有经验的资深Android移动开发、Java开发、前端开发、对鸿蒙感兴趣以及转行人员
→ 鸿蒙全栈最新学习笔记 希望这一份鸿蒙学习文档能够给大家带来帮助
更多推荐
所有评论(0)