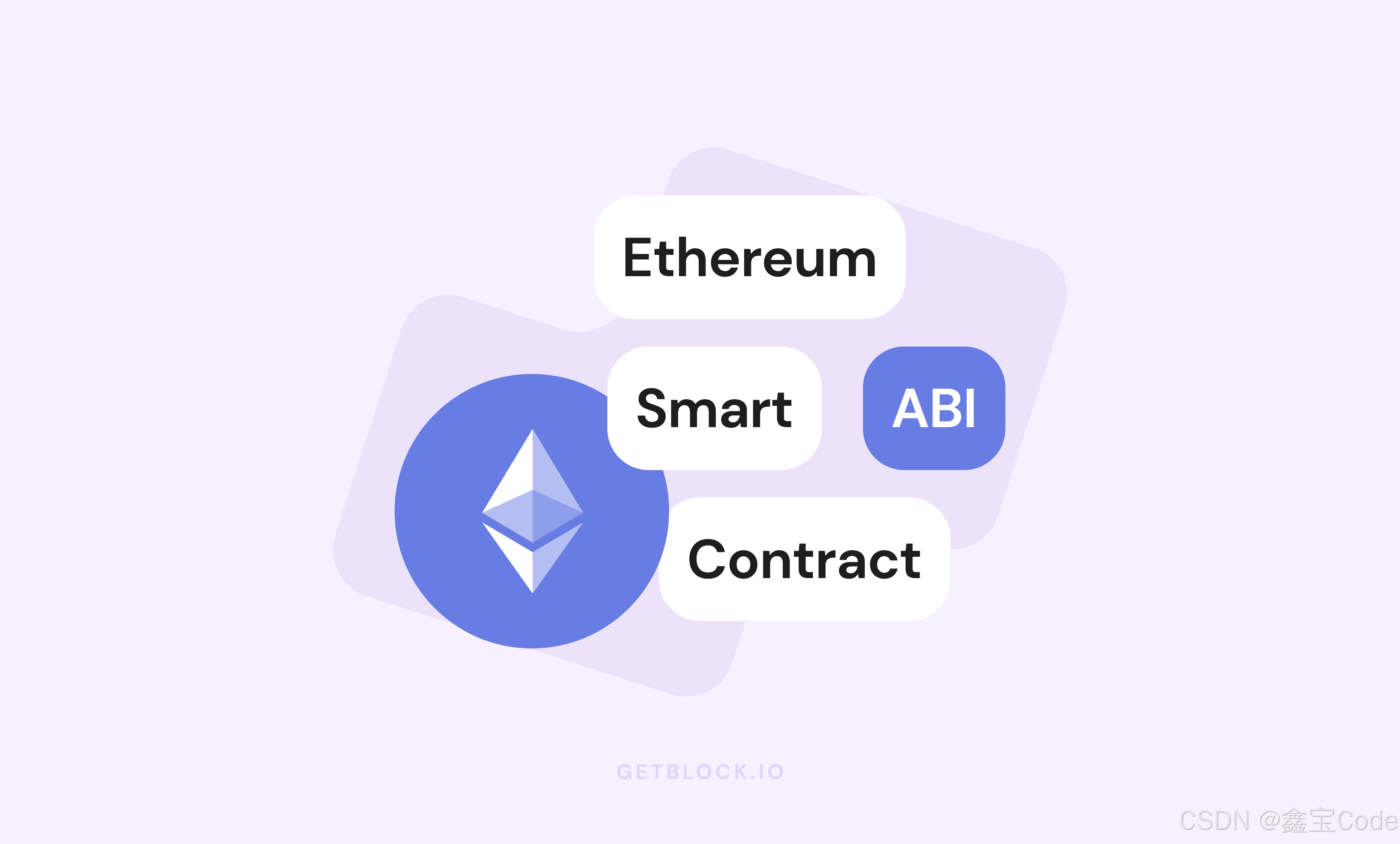
【区块链】深入理解智能合约 ABI
标准化接口定义确保交互的准确性提供跨平台兼容性支持合约升级和版本控制理解 ABI 的结构和格式掌握编码规则正确处理参数类型做好错误处理遵循最佳实践随着智能合约技术的发展,ABI 标准也在不断完善。开发者需要持续关注相关更新,以确保开发的应用程序能够稳定可靠地与智能合约进行交互。
·
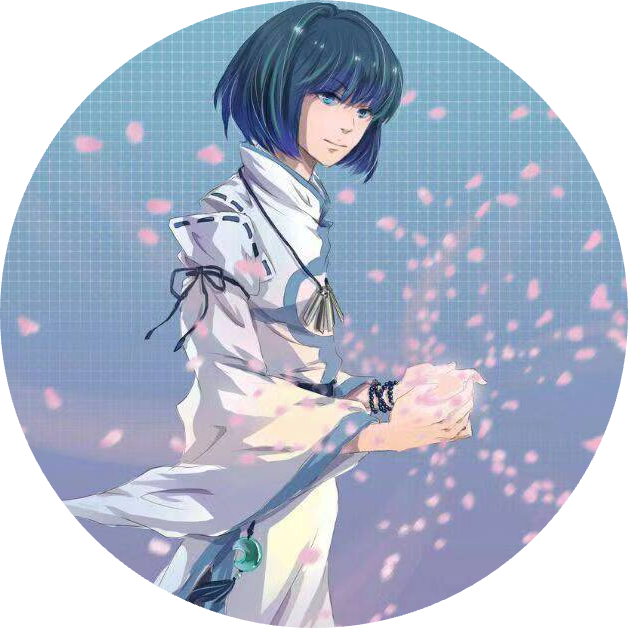

🌈个人主页: 鑫宝Code
🔥热门专栏: 闲话杂谈| 炫酷HTML | JavaScript基础
💫个人格言: "如无必要,勿增实体"
文章目录
深入理解智能合约 ABI(应用程序二进制接口)
一、ABI 基础概念
ABI(Application Binary Interface)是以太坊智能合约的接口标准,它定义了如何与智能合约进行交互的规范。ABI 是连接智能合约和外部世界的桥梁,使得应用程序能够正确地调用智能合约的函数并解析其返回值。
1.1 为什么需要 ABI?
- 智能合约部署后是二进制形式
- 需要标准化的接口定义
- 确保数据编码的一致性
- 实现跨平台调用
二、ABI 的结构和格式
2.1 基本结构示例
[
{
"type": "function",
"name": "transfer",
"inputs": [
{
"name": "recipient",
"type": "address"
},
{
"name": "amount",
"type": "uint256"
}
],
"outputs": [
{
"name": "",
"type": "bool"
}
],
"stateMutability": "nonpayable"
},
{
"type": "event",
"name": "Transfer",
"inputs": [
{
"name": "from",
"type": "address",
"indexed": true
},
{
"name": "to",
"type": "address",
"indexed": true
},
{
"name": "value",
"type": "uint256",
"indexed": false
}
],
"anonymous": false
}
]
2.2 主要组成部分
- 函数定义
- 事件定义
- 错误定义
- 构造函数定义
三、ABI 编码规则
3.1 函数选择器
// Solidity 合约
contract Example {
function transfer(address recipient, uint256 amount) public returns (bool) {
// 实现逻辑
}
}
// 函数选择器计算
// transfer(address,uint256) => 0xa9059cbb
3.2 参数编码
// Web3.js 中的参数编码示例
const web3 = new Web3();
const encodedParameters = web3.eth.abi.encodeParameters(
['address', 'uint256'],
['0x123...', '1000000000000000000']
);
四、实际应用示例
4.1 合约部署
// 智能合约
contract Token {
mapping(address => uint256) public balances;
function transfer(address to, uint256 amount) public returns (bool) {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
balances[to] += amount;
return true;
}
}
4.2 前端交互
// 使用 ethers.js 调用合约
const contract = new ethers.Contract(address, abi, provider);
// 调用合约函数
async function transferTokens(to, amount) {
try {
const tx = await contract.transfer(to, amount);
await tx.wait();
console.log('转账成功');
} catch (error) {
console.error('转账失败:', error);
}
}
五、ABI 的高级特性
5.1 函数重载
contract OverloadExample {
// ABI 会区分这两个函数
function getValue(uint256 id) public view returns (uint256) {}
function getValue(address addr) public view returns (uint256) {}
}
5.2 动态类型处理
contract DynamicExample {
// 动态数组参数
function processArray(uint256[] memory data) public {
// 处理逻辑
}
// 动态字符串
function setName(string memory name) public {
// 处理逻辑
}
}
六、ABI 工具和库
6.1 Web3.js 使用示例
// 编码函数调用
const web3 = new Web3();
const encodedCall = web3.eth.abi.encodeFunctionCall({
name: 'transfer',
type: 'function',
inputs: [{
type: 'address',
name: 'recipient'
}, {
type: 'uint256',
name: 'amount'
}]
}, ['0x123...', '1000000000000000000']);
6.2 Ethers.js 使用示例
// 使用 ethers.js 解析事件日志
const interface = new ethers.utils.Interface(abi);
const parsedLog = interface.parseLog(log);
console.log('事件名称:', parsedLog.name);
console.log('事件参数:', parsedLog.args);
七、常见问题和解决方案
7.1 ABI 解析错误
// ABI 验证函数
function validateABI(abi) {
try {
JSON.parse(JSON.stringify(abi));
return true;
} catch (error) {
console.error('ABI 格式无效:', error);
return false;
}
}
7.2 参数类型不匹配
// 参数类型检查
function validateParameter(type, value) {
switch(type) {
case 'address':
return web3.utils.isAddress(value);
case 'uint256':
return !isNaN(value) && value >= 0;
// 其他类型检查
default:
return true;
}
}
八、最佳实践
8.1 ABI 管理
// ABI 存储和版本控制
const contractABIs = {
v1: require('./abis/v1.json'),
v2: require('./abis/v2.json')
};
function getContractABI(version) {
return contractABIs[version] || contractABIs.v1;
}
8.2 错误处理
async function safeContractCall(contract, method, ...args) {
try {
const result = await contract[method](...args);
return { success: true, data: result };
} catch (error) {
console.error(`合约调用失败: ${method}`, error);
return { success: false, error };
}
}
九、总结
ABI 是智能合约开发中不可或缺的组成部分,它的重要性体现在:
- 标准化接口定义
- 确保交互的准确性
- 提供跨平台兼容性
- 支持合约升级和版本控制
关键要点:
- 理解 ABI 的结构和格式
- 掌握编码规则
- 正确处理参数类型
- 做好错误处理
- 遵循最佳实践
随着智能合约技术的发展,ABI 标准也在不断完善。开发者需要持续关注相关更新,以确保开发的应用程序能够稳定可靠地与智能合约进行交互。
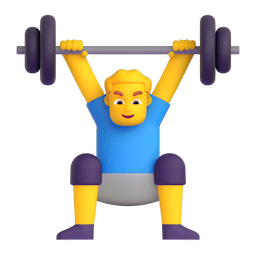
更多推荐
所有评论(0)