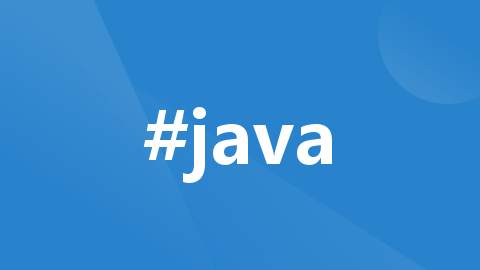
SpringBoot,SpringCloudAlibaba,GateWay,Nacos,OpenFeign,Vue
SpringBoot,SpringCloudAlibaba,GateWay,Nacos,OpenFeign,Vue
·
springboot,vue,springcloudalibaba课程视频,有需要可以看看
<!-- springboot,springboot整合redis,整合rocketmq视频: -->
https://www.bilibili.com/video/BV1nkmRYSErk/?vd_source=14d27ec13a4737c281b7c79463687112
<!-- springcloudalibaba,openfeign,nacos,gateway,sso视频:-->
https://www.bilibili.com/video/BV1cFDEYWEkY/?vd_source=14d27ec13a4737c281b7c79463687112
<!-- vue+springboot前后端分离视频:-->
https://www.bilibili.com/video/BV1JLSEYJETc/?vd_source=14d27ec13a4737c281b7c79463687112
<!-- shiro视频:-->
https://www.bilibili.com/video/BV1YVUmYJEPi/?vd_source=14d27ec13a4737c281b7c79463687112
Spring Cloud Alibaba 提供了一系列组件,以下是主要的组件及其简要说明:
组件 | 说明 |
---|---|
Nacos | 服务注册和配置中心,提供动态服务发现、配置管理和服务管理功能。 |
Sentinel | 熔断限流组件,提供流量控制、熔断降级和系统负载保护等功能。 |
Seata | 分布式事务解决方案,支持高性能和易于使用的分布式事务处理。 |
RocketMQ | 消息队列组件,提供低延迟、高可靠、万亿级容量的消息传递能力。 |
Dubbo | 高性能的Java RPC框架,用于服务间的调用。 |
Alibaba Cloud OSS | 阿里云对象存储服务,提供安全、低成本、高可靠的云存储服务。 |
Alibaba Cloud SchedulerX | 分布式任务调度产品,提供秒级、精准、高可靠、高可用的定时任务调度服务。 |
Alibaba Cloud SMS | 覆盖全球的短信服务,提供友好、高效、智能的通讯能力。 |
完整案例
好的,下面是一个使用Spring Cloud Alibaba的完整案例,其中包含两个微服务:user-service
(用户服务)和order-service
(订单服务),以及一个gateway
(网关)。order-service
将调用user-service
。我们将使用Nacos作为服务注册和配置中心,使用OpenFeign进行服务间调用。
1. Nacos Server
首先,你需要一个Nacos服务器。可以从Nacos官网下载并启动Nacos服务器。
2. user-service(用户服务)
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
</dependencies>
application.yml
server:
port: 8001
spring:
application:
name: user-service
cloud:
nacos:
discovery:
server-addr: localhost:8848
UserApplication.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class UserApplication {
public static void main(String[] args) {
SpringApplication.run(UserApplication.class, args);
}
}
User.java
public class User {
private Long id;
private String name;
// Getters and Setters
}
UserService.java
import org.springframework.stereotype.Service;
@Service
public class UserService {
public User getUserById(Long id) {
// Simulate database retrieval
return new User(id, "User" + id);
}
}
UserController.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userService.getUserById(id);
}
}
3. order-service(订单服务)
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
</dependencies>
application.yml
server:
port: 8002
spring:
application:
name: order-service
cloud:
nacos:
discovery:
server-addr: localhost:8848
OrderApplication.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
@SpringBootApplication
@EnableDiscoveryClient
@EnableFeignClients
public class OrderApplication {
public static void main(String[] args) {
SpringApplication.run(OrderApplication.class, args);
}
}
UserClient.java
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@FeignClient(value = "user-service")
public interface UserClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable Long id);
}
OrderService.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
@Autowired
private UserClient userClient;
public String createOrderForUser(Long userId) {
User user = userClient.getUserById(userId);
return "Order created for user " + user.getName();
}
}
OrderController.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class OrderController {
@Autowired
private OrderService orderService;
@GetMapping("/orders/{userId}")
public String createOrder(@PathVariable Long userId) {
return orderService.createOrderForUser(userId);
}
}
4. gateway(网关)
pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
application.yml
server:
port: 9999
spring:
application:
name: gateway
cloud:
nacos:
discovery:
server-addr: localhost:8848
gateway:
routes:
- id: user-service-route
uri: lb://user-service
predicates:
- Path=/user/**
filters:
- StripPrefix=1
- id: order-service-route
uri: lb://order-service
predicates:
- Path=/order/**
filters:
- StripPrefix=1
GatewayApplication.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
启动顺序
- 启动Nacos服务器。
- 启动
user-service
。 - 启动
order-service
。 - 启动
gateway
。 - 测试访问:
- 访问
http://localhost:9999/user/users/1
,将被转发到user-service
。 - 访问
http://localhost:9999/order/orders/1
,将被转发到order-service
,order-service
会调用user-service
。
这样,我们就通过Spring Cloud Gateway实现了对user-service
和order-service
的统一路由管理,并且order-service
可以通过OpenFeign调用user-service
。
更多推荐
所有评论(0)