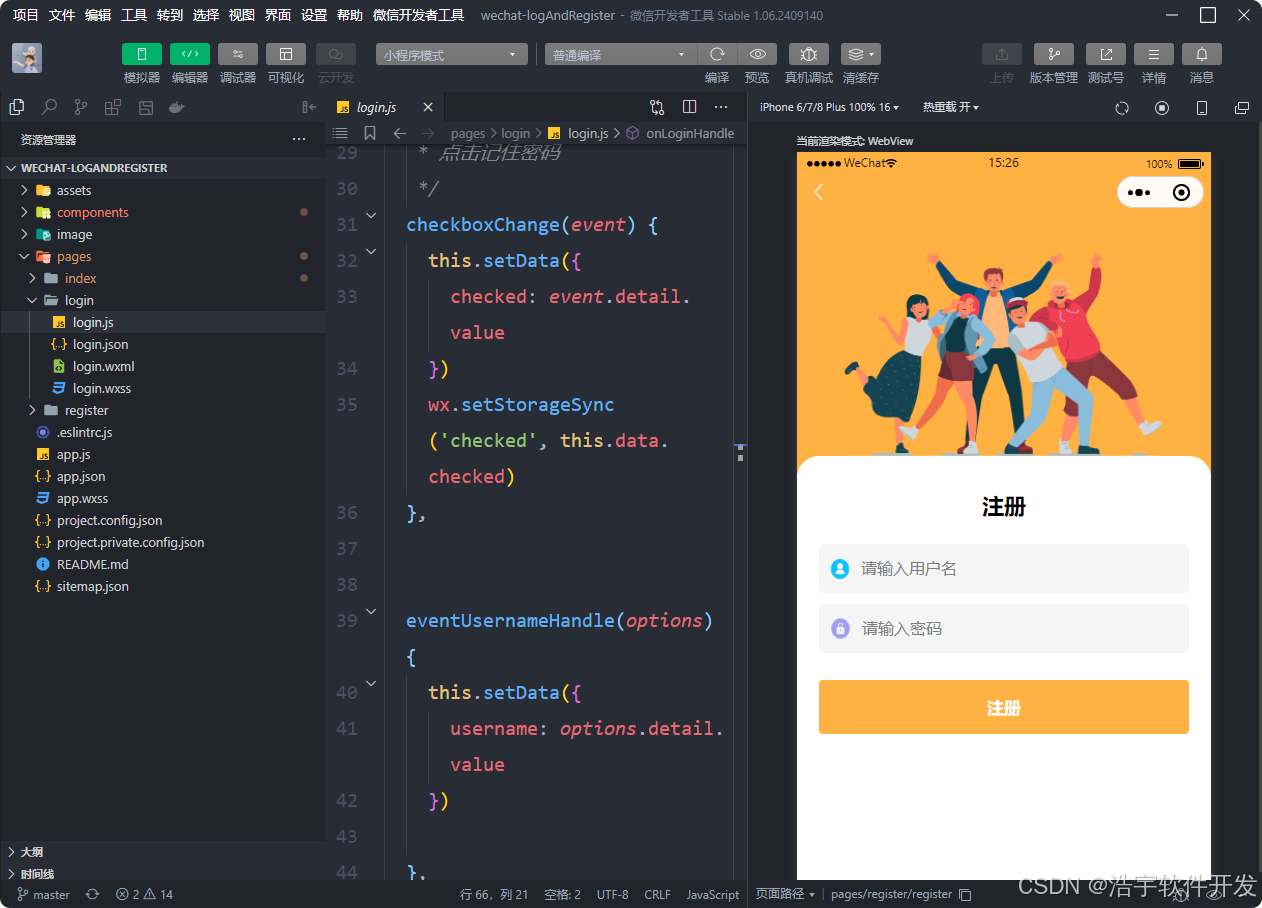
微信小程序实现登录注册
本人在b站录制的一些视频教程项目,免费供大家学习。
·
1. 官方文档教程
- https://developers.weixin.qq.com/miniprogram/dev/framework/
- 路由跳转的几种方式: https://developers.weixin.qq.com/miniprogram/dev/api/route/wx.switchTab.html
- Toast弹框提示:https://developers.weixin.qq.com/miniprogram/dev/api/ui/interaction/wx.showToast.html
- 数据缓存:https://developers.weixin.qq.com/miniprogram/dev/api/storage/wx.setStorageSync.html
2. 注册实现
- 编写register.wxml布局页面
<!--pages/register/register.wxml-->
<navigation-bar title="" back="{{true}}" color="white" background="#ffb241"/>
<view class="login-container">
<view class="logo-container">
<image class="logo" src="../../assets/img_login_bg.png" mode="" />
</view>
<view class="login-parent-container">
<text class="login-title-tips">注册</text>
<view class="login-username-container">
<image src="../../assets/img_username.png" mode="" />
<input placeholder="请输入用户名" bindinput="eventUsernameHandle" />
</view>
<view class="login-password-container">
<image src="../../assets/img_password.png" mode="" />
<input placeholder="请输入密码" password="true" bindinput="eventPasswordHandle" />
</view>
<view class="login-btn-container"> <button bind:tap="onRegisterHandle">注册</button></view>
</view>
</view>
navigation-bar是微信创建项目只带的顶部导航栏,直接拿过来使用即可
注意事项:
自定义组件需要注册之后才能使用, 所以需要在register.json文件中,注册自定义组件
{
"usingComponents": {
"navigation-bar": "/components/navigation-bar/navigation-bar"
}
}
- 编写register.wxss样式文件
/* pages/register/register.wxss */
.login-container {
display: flex;
height: 100%;
flex-direction: column;
background-color: #ffb241;
}
.logo-container {
display: flex;
width: 100%;
height: 436rpx;
justify-content: center;
background-color: #ffb241;
}
.logo-container .logo {
width: 648rpx;
height: 436rpx;
}
.login-parent-container {
display: flex;
flex-direction: column;
height: 100%;
background-color: #ffffff;
border-top-left-radius: 40rpx;
border-top-right-radius: 40rpx;
padding: 40rpx;
}
.login-title-tips {
display: flex;
width: 100%;
justify-content: center;
font-weight: 800;
font-size: 40rpx;
line-height: 100rpx;
}
.login-username-container {
display: flex;
flex-direction: row;
height: 90rpx;
align-items: center;
padding-left: 20rpx;
padding-right: 20rpx;
background-color: #f5f5f5;
border-radius: 10rpx;
margin-top: 20rpx;
}
.login-username-container image {
width: 40rpx;
height: 40rpx;
}
.login-username-container input {
width: 100%;
margin-left: 20rpx;
width: calc(100% - 60rpx);
}
.login-password-container {
display: flex;
flex-direction: row;
height: 90rpx;
align-items: center;
padding-left: 20rpx;
padding-right: 20rpx;
background-color: #f5f5f5;
border-radius: 10rpx;
margin-top: 20rpx;
}
.login-password-container image {
width: 40rpx;
height: 40rpx;
}
.login-password-container input {
width: 100%;
margin-left: 20rpx;
width: calc(100% - 60rpx);
}
.forget-pwd-right-container .tips {
font-size: 22rpx;
}
.forget-pwd-right-container .register-text {
font-size: 22rpx;
color: #ffb241;
}
.login-btn-container {
margin-top: 50rpx;
display: flex;
}
.login-btn-container button {
width: 100%;
line-height: 70rpx;
background-color: #ffb241;
color: #ffffff;
}
- register.js 实现注册
在这里使用wx.setStorageSync
来保存用户名和密码
// pages/register/register.js
Page({
/**
* 页面的初始数据
*/
data: {
username: "",
password: ""
},
/**
* 生命周期函数--监听页面加载
*/
onLoad(options) {
},
eventUsernameHandle(options) {
this.setData({
username: options.detail.value
})
},
eventPasswordHandle(options) {
this.setData({
password: options.detail.value
})
},
/**
* 注册
*/
onRegisterHandle() {
if (this.data.username.trim() === '') {
wx.showToast({
title: '请输入用户名',
icon: "error"
})
return
}
if (this.data.password.trim() === '') {
wx.showToast({
title: '请输入密码',
icon: "error"
})
return
}
//保存用户名和密码
wx.setStorageSync('username', this.data.username)
wx.setStorageSync('password', this.data.password)
wx.showToast({
title: '注册成功',
icon: 'success',
success: () => {
setTimeout(() => {
wx.navigateBack()
}, 1000);
}
})
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady() {
},
/**
* 生命周期函数--监听页面显示
*/
onShow() {
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide() {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload() {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh() {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom() {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage() {
}
})
- 实现效果
3. 登录实现
- 编写login.wxml
<!--pages/login/login.wxml-->
<navigation-bar title="" back="{{false}}" color="#ffffff" background="#ffb241" />
<view class="login-container">
<view class="logo-container">
<image class="logo" src="../../assets/img_login_bg.png" mode="" />
</view>
<view class="login-parent-container">
<text class="login-title-tips">登录</text>
<view class="login-username-container">
<image src="../../assets/img_username.png" mode="" />
<input placeholder="请输入用户名" bindinput="eventUsernameHandle" value="{{username}}" />
</view>
<view class="login-password-container">
<image src="../../assets/img_password.png" mode="" />
<input placeholder="请输入密码" password="true" bindinput="eventPasswordHandle" value="{{password}}" />
</view>
<view class="forget-pwd-container">
<view class="forget-pwd-left-container">
<switch type="checkbox" checked="{{checked}}" bindchange="checkboxChange" />
<text>记住密码</text>
</view>
<view class="forget-pwd-right-container" bind:tap="onRegisterHandle">
<text class="tips">还没有账号?</text>
<text class="register-text">注册</text>
</view>
</view>
<view class="login-btn-container"> <button bind:tap="onLoginHandle">登录</button></view>
</view>
</view>
- 编写login.wxss样式文件
/* pages/login/login.wxss */
.login-container {
display: flex;
height: 100%;
flex-direction: column;
background-color: #ffb241;
}
.logo-container {
display: flex;
width: 100%;
height: 436rpx;
justify-content: center;
background-color: #ffb241;
}
.logo-container .logo {
width: 648rpx;
height: 436rpx;
}
.login-parent-container {
display: flex;
flex-direction: column;
height: 100%;
background-color: #ffffff;
border-top-left-radius: 40rpx;
border-top-right-radius: 40rpx;
padding: 40rpx;
}
.login-title-tips {
display: flex;
width: 100%;
justify-content: center;
font-weight: 800;
font-size: 40rpx;
line-height: 100rpx;
}
.login-username-container {
display: flex;
flex-direction: row;
height: 90rpx;
align-items: center;
padding-left: 20rpx;
padding-right: 20rpx;
background-color: #f5f5f5;
border-radius: 10rpx;
margin-top: 20rpx;
}
.login-username-container image {
width: 40rpx;
height: 40rpx;
}
.login-username-container input {
width: calc(100% - 60rpx);
margin-left: 20rpx;
}
.login-password-container {
display: flex;
flex-direction: row;
height: 90rpx;
align-items: center;
padding-left: 20rpx;
padding-right: 20rpx;
background-color: #f5f5f5;
border-radius: 10rpx;
margin-top: 20rpx;
}
.login-password-container image {
width: 40rpx;
height: 40rpx;
}
.login-password-container input {
width: calc(100% - 60rpx);
margin-left: 20rpx;
}
.forget-pwd-container {
margin-top: 30rpx;
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
}
.forget-pwd-container .forget-pwd-left-container {
display: flex;
flex-direction: row;
align-items: center;
}
.forget-pwd-container .forget-pwd-right-container {
display: flex;
flex-direction: row;
}
.forget-pwd-right-container .tips {
font-size: 22rpx;
}
.forget-pwd-right-container .register-text {
font-size: 22rpx;
color: #ffb241;
}
.login-btn-container {
margin-top: 50rpx;
display: flex;
}
.login-btn-container button {
width: 100%;
line-height: 70rpx;
background-color: #ffb241;
color: #ffffff;
}
- login.js 实现登录
// pages/login/login.js
Page({
/**
* 页面的初始数据
*/
data: {
username: "",
password: "",
checked: false
},
/**
* 生命周期函数--监听页面加载
*/
onLoad(options) {
if (wx.getStorageSync("checked")) {
this.setData({
username: wx.getStorageSync("username"),
password: wx.getStorageSync("password"),
})
this.setData({checked:wx.getStorageSync('checked')})
}
},
/**
* 点击记住密码
*/
checkboxChange(event) {
this.setData({
checked: event.detail.value
})
wx.setStorageSync('checked', this.data.checked)
},
eventUsernameHandle(options) {
this.setData({
username: options.detail.value
})
},
eventPasswordHandle(options) {
this.setData({
password: options.detail.value
})
},
/**
* 注册
*/
onLoginHandle() {
if (this.data.username.trim() === '') {
wx.showToast({
title: '请输入用户名',
icon: "error"
})
return
}
if (this.data.password.trim() === '') {
wx.showToast({
title: '请输入密码',
icon: "error"
})
return
}
if (this.data.username === wx.getStorageSync("username") && this.data.password === wx.getStorageSync("password")) {
wx.showToast({
title: '登录成功',
icon: 'success',
success: () => {
setTimeout(() => {
wx.navigateBack()
}, 1000);
}
})
} else {
wx.showToast({
title: '用户名或密码错误',
icon: 'error',
})
}
},
/**
* 注册
*/
onRegisterHandle() {
wx.navigateTo({
url: '/pages/register/register',
})
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady() {
},
/**
* 生命周期函数--监听页面显示
*/
onShow() {
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide() {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload() {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh() {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom() {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage() {
}
})
- 运行效果
4. 关于作者其它项目视频教程介绍
本人在b站录制的一些视频教程项目,免费供大家学习
- Android新闻资讯app实战:https://www.bilibili.com/video/BV1CA1vYoEad/?vd_source=984bb03f768809c7d33f20179343d8c8
- Androidstudio开发购物商城实战:https://www.bilibili.com/video/BV1PjHfeXE8U/?vd_source=984bb03f768809c7d33f20179343d8c8
- Android开发备忘录记事本实战:https://www.bilibili.com/video/BV1FJ4m1u76G?vd_source=984bb03f768809c7d33f20179343d8c8&spm_id_from=333.788.videopod.sections
- Androidstudio底部导航栏实现:https://www.bilibili.com/video/BV1XB4y1d7et/?spm_id_from=333.337.search-card.all.click&vd_source=984bb03f768809c7d33f20179343d8c8
- Android使用TabLayout+ViewPager2实现左右滑动切换:https://www.bilibili.com/video/BV1Mz4y1c7eX/?spm_id_from=333.337.search-card.all.click&vd_source=984bb03f768809c7d33f20179343d8c8
更多推荐
所有评论(0)