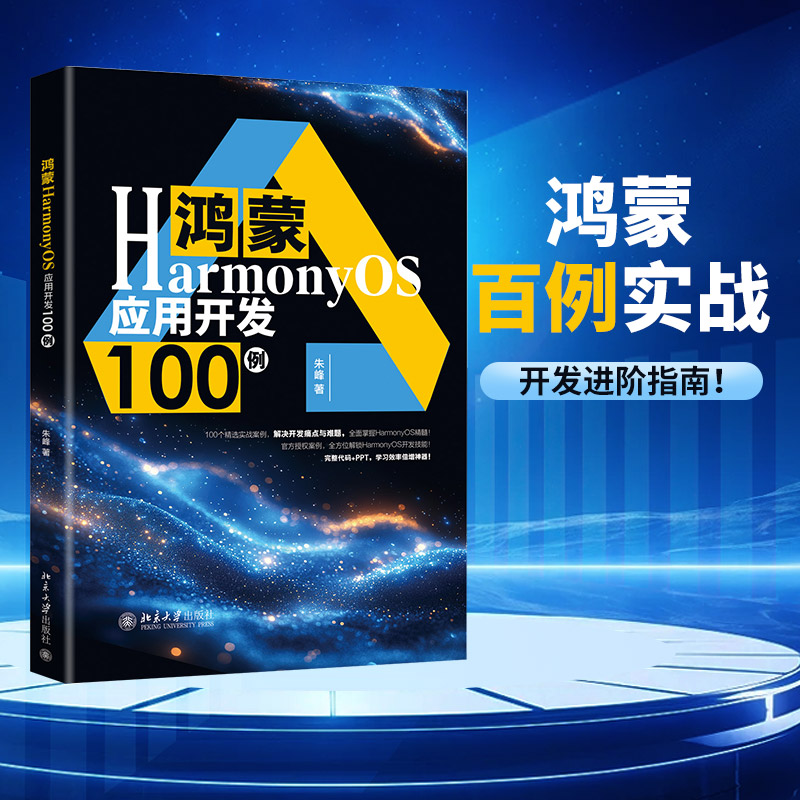
鸿蒙HarmonyOS应用开发100例实战指南
通过这100个真实案例,您将全面掌握鸿蒙应用开发的核心技能,快速构建跨设备、智能化的全场景应用!
·
真实案例解析:从基础到高阶的鸿蒙开发全攻略
核心卖点
- 100+真实场景案例:覆盖UI组件到AI智能交互全流程,提供可直接复用的完整代码
- HarmonyOS核心技术解密:分布式架构、ArkUI 3.0与AI Kit深度整合
- 全场景开发方案:手机、智慧屏、车机等多设备适配实战
- 全方位学习资源:完整代码+配套PPT+专属视频教程三位一体
精选案例代码示例
案例1:ArkUI基础组件开发 - 个性化按钮
// 自定义按钮组件
@Component
struct CustomButton {
@Prop text: string = '按钮'
@Prop backgroundColor: Color = Color.Blue
@State isPressed: boolean = false
build() {
Button(this.text)
.width(150)
.height(50)
.backgroundColor(this.isPressed ? this.backgroundColor : Color.Gray)
.onClick(() => {
console.log('按钮被点击')
})
.onTouch((event: TouchEvent) => {
if (event.type === TouchType.Down) {
this.isPressed = true
} else if (event.type === TouchType.Up) {
this.isPressed = false
}
})
}
}
// 使用示例
@Entry
@Component
struct ButtonExample {
build() {
Column({ space: 10 }) {
CustomButton({ text: '主要按钮', backgroundColor: Color.Blue })
CustomButton({ text: '成功按钮', backgroundColor: Color.Green })
CustomButton({ text: '警告按钮', backgroundColor: Color.Orange })
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
}
案例2:分布式能力 - 跨设备文件共享
import distributedFile from '@ohos.file.distributedFile';
import common from '@ohos.app.ability.common';
@Entry
@Component
struct FileShareExample {
@State devices: Array<string> = []
@State progress: number = 0
// 发现附近设备
discoverDevices() {
try {
let context = getContext(this) as common.UIAbilityContext
distributedFile.startDiscovering(context, (deviceList) => {
this.devices = deviceList
})
} catch (error) {
console.error('设备发现失败:', error)
}
}
// 发送文件到指定设备
async sendFile(deviceId: string) {
let context = getContext(this) as common.UIAbilityContext
let srcPath = context.filesDir + '/example.txt'
try {
let file = await distributedFile.createDistributedFile(srcPath)
await file.transfer(deviceId, (transferred: number, total: number) => {
this.progress = Math.round((transferred / total) * 100)
})
console.info('文件传输完成')
} catch (error) {
console.error('文件传输失败:', error)
}
}
build() {
Column() {
Button('扫描附近设备')
.onClick(() => this.discoverDevices())
.margin(10)
List({ space: 10 }) {
ForEach(this.devices, (device) => {
ListItem() {
Row() {
Text(device)
Button('发送文件')
.onClick(() => this.sendFile(device))
}
}
})
}
.width('90%')
Text(`传输进度: ${this.progress}%`)
.fontSize(20)
}
.width('100%')
.height('100%')
}
}
案例3:AI能力集成 - 图像识别
import image from '@ohos.multimedia.image';
import ai from '@ohos.ai';
@Entry
@Component
struct ImageRecognition {
@State result: string = '点击识别按钮开始分析'
@State imageSrc: Resource = $r('app.media.example')
// 图像识别函数
async recognizeImage() {
try {
// 1. 获取图像像素数据
let imageSource = image.createImageSource(this.imageSrc)
let imagePixel = await imageSource.createPixelMap()
// 2. 初始化AI引擎
let context = getContext(this) as common.UIAbilityContext
let aiEngine = await ai.createAiEngine(context)
// 3. 执行图像识别
let result = await aiEngine.executeImageRecognition(imagePixel)
// 4. 处理识别结果
this.result = `识别结果: ${result[0].name} (置信度: ${(result[0].confidence * 100).toFixed(1)}%)`
} catch (error) {
console.error('识别失败:', error)
this.result = '识别失败,请重试'
}
}
build() {
Column() {
Image(this.imageSrc)
.width(300)
.height(300)
.margin(10)
Button('识别图像')
.onClick(() => this.recognizeImage())
.width(200)
.margin(10)
Text(this.result)
.fontSize(18)
.margin(10)
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
}
内容架构
第一章:基本UI组件开发(20例)
- 按钮、文本、输入框等基础组件深度定制
- 列表、网格、卡片等布局组件实战
- 动画与交互动效实现
第二章:图形与图像开发(15例)
- 自定义绘图与图表实现
- 图像处理与滤镜效果
- 相机拍照与图片编辑
第三章:多媒体开发(15例)
- 音频播放与录制
- 视频播放器定制开发
- 媒体会话与通知控制
第四章:网络与数据管理(15例)
- HTTP/WebSocket通信
- 本地数据库操作
- 分布式数据管理
第五章:定位与地图开发(10例)
- 地理位置服务
- 地图集成与自定义标记
- 轨迹记录与分析
第六章:系统能力开发(15例)
- 传感器数据采集
- 设备信息获取
- 后台任务管理
第七章:AI开发(10例)
- 图像识别与分类
- 语音识别与合成
- 自然语言处理
配套资源说明
- 完整源代码:每个案例提供可直接运行的工程代码
- 详细注释:关键代码段配有中文注释说明
- 配套PPT:技术要点与实现思路总结
- 视频教程:扫码观看案例实现过程演示
本书适合:
- 已有HarmonyOS基础想进阶的开发者
- 需要快速实现特定功能的工程师
- 院校师生及培训机构教学参考
- 对全场景开发感兴趣的技术爱好者
通过这100个真实案例,您将全面掌握鸿蒙应用开发的核心技能,快速构建跨设备、智能化的全场景应用!
更多推荐
所有评论(0)