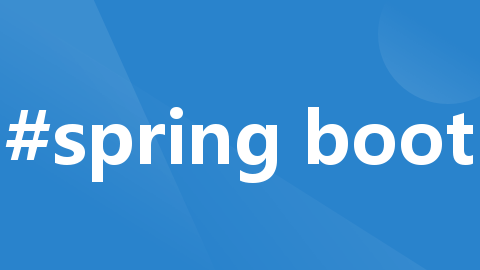
【SpringBoot】| Thymeleaf 模板引擎
【SpringBoot】| Thymeleaf 模板引擎
目录
Thymeleaf 模板引擎
Thymeleaf 是一个流行的模板引擎,该模板引擎采用 Java 语言开发。
模板引擎是一个技术名词,是跨领域跨平台的概念,在 Java 语言体系下有模板引擎,在 C#、PHP 语言体系下也有模板引擎,甚至在 JavaScript 中也会用到模板引擎技术,Java 生态下 的模板引擎有 Thymeleaf 、Freemaker、Velocity、Beetl(国产) 等。
Thymeleaf 对网络环境不存在严格的要求,既能用于 Web 环境下,也能用于非 Web 环境 下。在非 Web 环境下,他能直接显示模板上的静态数据;在 Web 环境下,它能像 Jsp 一样从 后台接收数据并替换掉模板上的静态数据。它是基于 HTML 的,以 HTML 标签为载体, Thymeleaf 要寄托在 HTML 标签下实现。
Spring Boot 集成了 Thymeleaf 模板技术,并且 Spring Boot 官方也推荐使用 Thymeleaf 来 替代 JSP 技术,Thymeleaf 是另外的一种模板技术,它本身并不属于 Spring Boot,Spring Boot 只是很好地集成这种模板技术,作为前端页面的数据展示,在过去的 Java Web 开发中,我们 往往会选择使用 Jsp 去完成页面的动态渲染,但是 jsp 需要翻译编译运行,效率低 。
Thymeleaf 的官方网站:Thymeleaf
Thymeleaf 官方手册:Tutorial: Using Thymeleaf
1. 第一个例子
①创建项目,引入web和thymeleaf依赖
②pom.xml主要依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.15</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.zl</groupId>
<artifactId>springboot-myredis</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-myredis</name>
<description>springboot-myredis</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!--模板依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!--web依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
③Controller编写存放数据
package com.zl.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import javax.servlet.http.HttpServletRequest;
@Controller
public class HelloThymeleafController {
@GetMapping("/hello")
public String helloThymeleafController(HttpServletRequest request){
// 添加数据到request域当中,模板引擎可以从request域当中获取
request.setAttribute("data","第一个Thymeleaf数据");
// 返回到视图(html)
return "hello";
}
}
④视图hello.html取出数据(放到templates中)
<!DOCTYPE html>
<!--加入xmlns命名空间-->
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<!--取出数据-->
<h1 th:text="${data}"></h1>想显示的数据
</body>
</html>
⑤进行访问
模板引擎的常用配置
思考:为什么controller返回一个字符串就可以直接找到templates下的对应html呢?这些和模板引擎的默认设置是有关的!
application.properties配置
注:在开发阶段只需要把cache设置为false即可,其它都是默认选项不需要修改!
#开发阶段设置为 false ,上线后设置为 true,默认是true走缓存
spring.thymeleaf.cache=false
# 设置模版文件的路径,前缀,默认就是classpath:/templates/
spring.thymeleaf.prefix=classpath:/templates/
# 设置后缀,默认就是 .html
spring.thymeleaf.suffix=.html
#编码方式,默认就是UTF-8,所以中文不会乱码
spring.thymeleaf.encoding=UTF-8
#模板的类型,默认是HTML,模板是html文件
spring.thymeleaf.mode=HTML
2. 表达式
表达式是在页面获取数据的一种 thymeleaf 语法。类似 ${key} 。
①标准变量表达式
注意:th:text="${key}" 是 Thymeleaf 的一个属性,用于文本的显示。
语法: ${key} 。
说明:标准变量表达式用于访问容器(tomcat)上下文环境中的变量,功能和 EL 中的 ${} 相 同。Thymeleaf 中的变量表达式使用 ${变量名} 的方式获取 Controller 中 model 其中的数据。 也就是 request 作用域中的数据。
准备Student数据
package com.zl.pojo;
public class Student {
private String name;
private Integer age;
public Student(String name, Integer age) {
this.name = name;
this.age = age;
}
public Student() {
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
欢迎页面index.html放入静态资源static下,不走模板引擎
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<a href="thymeleaf/expression1">标准变量表达式</a>
</body>
</html>
application.properties
#配置端口号
server.port=8082
#开发阶段设置为 false ,上线后设置为 true,默认是true走缓存
spring.thymeleaf.cache=false
controller存放数据,跳转到expression1
package com.zl.controller;
import com.zl.pojo.Student;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/expression1")
public String expression1(Model model){
// 添加数据
model.addAttribute("student",new Student("Jack",18));
// 跳转到视图
return "expression1";
}
}
expression1.html接收数据
注:${student.getName()}也能获取到值,底层实际上就是调用对应的Get方法!
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>expression1</title>
</head>
<body>
<h1 th:text="${student.name}"></h1>
<h1 th:text="${student.age}"></h1>
<!--调用g对应的get方法也可以-->
<h1 th:text="${student.getAge()}"></h1>
</body>
</html>
执行结果:
②选择变量表达式(星号变量表达式)
语法:*{key} 。
说明:不能单独使用,需要配和 th:object 一起使用。选择变量表达式,也叫星号变量表达式,使用 th:object 属性来绑定对象,选择表达式首先使用 th:object 来绑定后台传来的对象,然后使用 * 来代表这个对象,后面 {} 中的值是此对象中的属性。可以简化上述的开发。
欢迎页面
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<a href="thymeleaf/expression1">标准变量表达式</a>
<a href="thymeleaf/expression2">选择变量表达式</a>
</body>
</html>
controller存放数据
@GetMapping("/expression2")
public String expression2(Model model){
model.addAttribute("student",new Student("Jack",18));
return "expression2";
}
expression2.html取出数据:使用th:object +*{key}简化上述的开发
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>expression2</title>
</head>
<body>
<!--前缀-->
<div th:object="${student}">
<!--属性-->
<p th:text="*{name}"></p>
<p th:text="*{age}"></p>
</div>
<!--实际上使用*{}也可以完整的表示对象的属性值,和${}效果一样-->
<p th:text="*{student.name}"></p>
</body>
</html>
③链接表达式(URL表达式)
语法:@{链接 url} 。
说明:主要用于链接、地址的展示,可用于 <script src="..."> , <link href="..."> <a href=".."> ,<form action="..."> <img src="...">等,可以在 URL 路径中动态获取数据 。
欢迎页面index.html
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<a href="thymeleaf/expression1">标准变量表达式</a>
<a href="thymeleaf/expression2">选择变量表达式</a>
<a href="thymeleaf/expression3">链接表达式</a>
</body>
</html>
controller测试
package com.zl.controller;
import com.zl.pojo.Student;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/expression1")
public String expression1(Model model){
model.addAttribute("student",new Student("Jack",18));
return "expression1";
}
@GetMapping("/expression2")
public String expression2(Model model){
model.addAttribute("student",new Student("Jack",18));
return "expression2";
}
// 链接表达式
@GetMapping("/expression3")
public String expression3(Model model){
model.addAttribute("userId",1001);
model.addAttribute("userName","Jack");
return "expression3";
}
// 测试连接表达式
@GetMapping("/query1")
@ResponseBody
public String query1(){ // 无参
return "id=";
}
@GetMapping("/query2")
@ResponseBody
public String query2(Integer id){ // 一个参数
return "id="+id;
}
@GetMapping("/query3")
@ResponseBody
public String query3(Integer id,String name){
return "id="+id+",name="+name;
}
}
expression3.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>expression3</title>
</head>
<body>
<!--使用绝对路径-->
<a th:href="@{http://www.baidu.com}">链接到百度</a>
<!--使用相对路径-->
<a th:href="@{/thymeleaf/query1}">测试无参链接表达式</a>
<!--使用字符串拼接的方式-->
<a th:href="@{'/thymeleaf/query2?id='+${userId}}">测试一个参数的链接表达式</a>
<!--常用的传参方式-->
<a th:href="@{/thymeleaf/query2(id=${userId})}">常用的传参方式</a>
<a th:href="@{/thymeleaf/query3(id=${userId},name=${userName})}">测试两个参数的链接表达式</a>
</body>
</html>
3. Thymeleaf的属性
大部分属性和 html 的一样,只不过前面加了一个 th 前缀。 加了 th 前缀的属性,是经过模版引擎处理的。 在属性上也可以使用变量表达式!
①th:action
定义后台控制器的路径,类似标签的 action 属性,主要结合 URL 表达式,获取动态变量
<form id="login" th:action="@{/login}" th:method="post">......</form>
②th:method
设置请求方法
<form id="login" th:action="@{/login}" th:method="${methodAttr}"></form>
③th:href
定义超链接,主要结合 URL 表达式,获取动态变量
<a th:href="@{/query/student}">相对地址没有传参数</a>
④th:src
用于外部资源引入,比如<script>标签的 src 属性,<img>标签的 src 属性,常与@{}表达式 结合使用,在 SpringBoot 项目的静态资源都放到 resources 的 static 目录下,放到 static 路径 下的内容,写路径时不需要写上 static
<script type="text/javascript" th:src="@{/js/jquery-3.4.1.js}"></script>
⑤th:text
用于文本的显示,该属性显示的文本在标签体中,如果是文本框,数据会在文本框外显示, 要想显示在文本框内,使用 th:value
<input type="text" id="realName" name="reaName" th:text="${realName}">
⑥th:style
设置样式
<a th:onclick="'fun1('+${user.id}+')'" th:style="'color:red'">点击我</a>
例1:
第一步:欢迎页面
<a href="thymeleaf/property">模板的属性</a>
第二步:controller准备数据
package com.zl.controller;
import com.zl.pojo.Student;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/property")
public String useProperty(Model model){
model.addAttribute("methodAttr","post");
model.addAttribute("id","123");
model.addAttribute("userName","Jack");
model.addAttribute("userValue","lisi");
return "html-property";
}
}
第三步:html-property.html获取数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>模板的属性</title>
</head>
<body>
<form th:action="@{/loginServet}" th:method="${methodAttr}">
<input th:type="text" th:name="${userName}" th:value="${userValue}">
<!--绑定按钮事件-->
<input th:type="button" id="btn" th:onclick="btnOnclick()" value="按钮">
</form>
<script type="text/javascript">
function btnOnclick() {
alert("按钮单击了");
}
</script>
</body>
</html>
F12查看
⑦th:each (重点)
这个属性非常常用,比如从后台传来一个对象集合那么就可以使用此属性遍历输出,它与 JSTL 中的<c:forEach>类似,此属性既可以循环遍历集合,也可以循环遍历数组及 Map!
例2:循环list集合或数组
第一步:欢迎页面
<a href="thymeleaf/eachList">循环遍历List</a>
第二步:controller准备数据
package com.zl.controller;
import com.zl.pojo.Student;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.ArrayList;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/eachList")
public String eachList(Model model){
// 准备数据
ArrayList<Student> studentList = new ArrayList<>();
Student s1 = new Student("zhangsan", 18);
Student s2 = new Student("lisi", 20);
Student s3 = new Student("wangwu", 22);
studentList.add(s1);
studentList.add(s2);
studentList.add(s3);
// 放入域当中
model.addAttribute("students",studentList);
return "student-list";
}
}
第三步:student-list.html获取数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>eachList</title>
</head>
<body>
<table border="1px" th:align="center" width="50%">
<tr>
<th>姓名</th>
<th>年龄</th>
</tr>
<tr th:each="stu:${students}">
<td th:text="${stu.name}"></td>
<td th:text="${stu.age}"></td>
</tr>
</table>
</body>
</html>
补充:实际上完整的遍历格式如下:
<div th:each="集合循环成员,循环的状态变量:${key}">
<p th:text="${集合循环成员}" ></p>
</div>
循环的状态变量,可以定义也可以不定义,通过该变量可以获取如下信息 :
①index: 当前迭代对象的 index(从 0 开始计算);
②count: 当前迭代对象的个数(从 1 开始计算)这两个用的较多;
③size: 被迭代对象的大小;
④current: 当前迭代变量,stuState.current就相当于循环变量stu;
⑤even/odd: 布尔值,当前循环是否是偶数/奇数(从 0 开始计算);
⑥first: 布尔值,当前循环是否是第一个;
⑦last: 布尔值,当前循环是否是最后一个;
例如:获取当前迭代对象的个数
注意:循环体信息 userStat 也可以不定义,则默认采用迭代变量加上 Stat 后缀,即
userStat。
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>eachList</title>
</head>
<body>
<table border="1px" th:align="center" width="50%">
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<!--添加状态变量stuState-->
<tr th:each="stu,stuState:${students}">
<td th:text="${stuState.count}"></td>
<td th:text="${stu.name}"></td>
<td th:text="${stu.age}"></td>
</tr>
</table>
</body>
</html>
执行结果
例3:循环map集合
以key和value的方式存放数据
@GetMapping("/eachMap")
public String eacMap(Model model){
// 准备数据
HashMap<String, Student> studentHashMap = new HashMap<>();
Student s1 = new Student("zhangsan", 18);
Student s2 = new Student("lisi", 20);
Student s3 = new Student("wangwu", 22);
studentHashMap.put("s1",s1);
studentHashMap.put("s2",s2);
studentHashMap.put("s3",s3);
// 放入域当中
model.addAttribute("studentHashMap",studentHashMap);
return "student-map";
}
取出数据
①调用循环变量的key属性拿到Map集合的key;
②调用循环变量的value属性拿到Map集合的value,此时拿到的value是Java对象,可以继续点下去取里面的成员变量属性;
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>eachList</title>
</head>
<body>
<div style="margin-left: 400px">
<div th:each="stuMap:${studentHashMap}">
<p th:text="${stuMap.key}"></p>
<p th:text="${stuMap.value}"></p>
<p th:text="${stuMap.value.name}"></p>
<p th:text="${stuMap.value.age}"></p>
<hr th:color="red">
</div>
</div>
</body>
</html>
执行结果:
例4:List<Map>复杂的List和Map集合的嵌套
存入两个map集合的数据到list集合
@GetMapping("/eachListMap")
public String eachListMap(Model model){
// 准备一个listmap
List<Map<String,Student>> listmap = new ArrayList<>();
// 第一个map1数据
HashMap<String, Student> map1 = new HashMap<>();
Student s1 = new Student("zhangsan", 18);
Student s2 = new Student("lisi", 20);
map1.put("s1",s1);
map1.put("s2",s2);
// 第二个map2数据
HashMap<String, Student> map2 = new HashMap<>();
Student s3 = new Student("Jack", 22);
Student s4 = new Student("Rose", 22);
map2.put("s3",s3);
map2.put("s4",s4);
// 把map集合放入list集合当中
listmap.add(map1);
listmap.add(map2);
// 放入域当中
model.addAttribute("listmap",listmap);
return "student-listmap";
}
两层each循环取出数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>eachList</title>
</head>
<body>
<div style="margin-left: 400px">
<div th:each="stuListMap:${listmap}"><!--得到每个map-->
<!--再循环一层,得到每个map的key和value-->
<div th:each="map:${stuListMap}">
<p th:text="${map.key}"></p>
<p th:text="${map.value}"></p>
</div>
<hr th:color="red">
</div>
</div>
</body>
</html>
执行结果:
⑧条件判断 if-unless
"th:if" : 判断语句,当条件为true,显示html标签体内,反之不显示 没有else语句。
语法:th:if=”boolean 条件” , 条件为 true 显示体内容
th:unless 是 th:if 的一个相反操作,条件为 false 显示体内容
第一步:index.html
<a href="thymeleaf/ifunless">判断语句if-unless</a><br>
第二步:controller发出请求
@GetMapping("/ifunless")
public String ifUnless(Model model){
// 有正常数据
model.addAttribute("sex","m");
// 数据为空字符串
model.addAttribute("name","");
// 数据为null
model.addAttribute("isOld",null);
return "if-unless";
}
第三步:前端进行判断
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>条件判断if-unless</title>
</head>
<body>
<div style="margin-left: 400px">
<p th:if="${sex == 'm'}">我是男孩子</p>
<!--空字符串是true-->
<p th:if="${name}">name是""</p>
<!--null是false-->
<p th:if="${isOld}">isOld是null</p>
<!--unless条件为false显示内容-->
<p th:unless="${isOld}">isOld是null------</p>
</div>
</body>
</html>
执行结果:
①if是条件为true显示结果,unless是条件为假显示结果;
②空字符串“”会被当做true来处理;
③nulll会被当做fale处理;
⑨switch-case 判断语句
语法:类似 java 中的 switch,case;最多只能有一个case匹配成功。
<div th:switch="要比对的值">
<p th:case="值1">
结果1
</p>
<p th:case="值2">
结果2
</p>
<p th:case="*">
默认结果
</p>
以上的case只有一个语句执行
</div>
controller存储数据
@GetMapping("/switchcase")
public String switchCase(Model model){
model.addAttribute("a","m");
model.addAttribute("b","f");
model.addAttribute("c","mf");
return "swicth-case";
}
html取出数据并判断
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>swicth-case条件判断</title>
</head>
<body>
<div style="margin-left: 400px">
<div th:switch="${a}">
<p th:case="m">男</p>
<p th:case="f">女</p>
<p th:case="*">未知</p>
</div>
<hr th:color="red">
<div th:switch="${b}">
<p th:case="m">男</p>
<p th:case="f">女</p>
<p th:case="*">未知</p>
</div>
<hr th:color="red">
<div th:switch="${c}">
<p th:case="m">男</p>
<p th:case="f">女</p>
<p th:case="*">未知</p>
</div>
</div>
</body>
</html>
执行结果:
总结:一旦某个 case 判断值为 true,剩余的 case 则都当做 false,“*”表示默认的case,前面的case 都不匹配时候,执行默认的 case。
⑩th:inline内联
th:inline 有三个取值类型 (text, javascript 和 none) !none就表示无的意思。
内联 text
可以让 Thymeleaf 表达式不依赖于 html 标签,直接使用内敛表达式[[表达式]]即可获取动 态数据,要求在父级标签上加 th:inline = “text”属性。
controller存储数据
package com.zl.controller;
import com.zl.pojo.Student;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.security.PublicKey;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/inline/text")
public String inlineText(Model model){
model.addAttribute("name","Jack");
model.addAttribute("age",22);
return "inline-text";
}
}
使用内联text取出数据
第一步:父级标签上加 th:inline = “text”;实际上这部分可以省略。
第二步:使用[[${变量的方式}]]。
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>inline-text</title>
</head>
<body>
<div style="margin-left: 400px" th:inline="text">
<!--使用html标签-->
<p th:text="${name}"></p><br>
<!--内联text-->
[[${age}]]
</div>
</body>
</html>
成功取出数据
内联javascript
就是在 js 中,获取模版中的数据!
使用内联javascript取出上述存取的数据
第一步:引入script标签,在标签中引入th:inline = “javascript”;
第二步:在js中取出数据,取出方式和内联text相同;
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>inline-text</title>
</head>
<body>
<!--按钮绑定点击事件-->
<button onclick="btnOnclick()">按钮</button>
<script type="text/javascript" th:inline="javascript">
/*使用js代码获取数据*/
var name = [[${name}]];
var age = [[${age}]];
<!--直接输出打印-->
alert("用户名"+name+",年龄"+age);
<!--绑定事件,使用回调函数-->
function btnOnclick(){
alert("用户名"+name+",年龄"+age);
}
</script>
</body>
</html>
执行结果
4. 字面量
①文本字面量,单引号'...'包围的字符串为文本字面量;
②数字字面量;
③boolean字面量;
④null字面量;
第一步:欢迎页面index.html
<a href="thymeleaf/text">字面量</a>
第二步:域中存数据
@GetMapping("/text")
public String text(Model model){
model.addAttribute("sex","m");
model.addAttribute("isLogin",true);
model.addAttribute("age",10);
model.addAttribute("name",null);
model.addAttribute("city","北京");
return "text";
}
第三步:取出数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>字面量</title>
</head>
<body>
<!--文本字面量,单引号''包裹的字符串-->
<p th:text="'城市是'+${city}"></p>
<!--文本字面量实际上使用内联也能完成-->
<div th:inline="text">
我的城市是[[${city}]]
</div>
<!--数字字面量-->
<p th:if="${age > 8}">age>8</p>
<!--boolean字面量-->
<p th:text="${isLogin == true}">登陆了</p>
<!--null字面量-->
<p th:if="${name == null}">name是null</p>
</body>
</html>
执行结果:
5.字符串连接(重要)
第一种方式: 使用 单引号括起来字符串 , 使用 + 连接其他的 字符串或者表达式;
<p th:text="'我是'+${name}+',我所在的城市'+${city}">数据显示</p>
第二种方式:使用双竖线||,例如:|字符串内容|;
<p th:text="|我是${name},我所在城市${city|">
显示数据
</p>
controllelr存储数据
@GetMapping("/stringbuf")
public String stringBuf(Model model){
model.addAttribute("name","Jack");
model.addAttribute("age",45);
model.addAttribute("student",new Student("张三",16));
return "stringbuf";
}
进行字符串的拼接
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<body>
<div style="margin-left: 400px">
<!--第一种方式-->
<p th:text=" '我是'+${name}+',今年'+${age}+',岁'+'带的学生信息是'+${student} "></p>\
<!--第二种方式-->
<hr style="color: chocolate">
<p th:text="|我是+${name},今年${age}岁,带的学生信息是${student}|"></p>
</div>
</body>
<head>
<meta charset="UTF-8">
<title>字符串的拼接</title>
</head>
</html>
执行结果
6. 运算符
①算术运 算: + , - , * , / , %
②关系比较 : > , < , >= , <= ( gt , lt , ge , le )
③相等判断: == , != ( eq , ne )④三元运算符:表达式 ? true的结果 : false的结果
controller存储数据
@GetMapping("/data")
public String data(Model model){
model.addAttribute("name1",null);
model.addAttribute("name2","Jack");
model.addAttribute("age",18);
model.addAttribute("isLogin",true);
return "data";
}
进行数据的运算符比较
注:对于内层嵌套的三元运算符,内层嵌套的不用${}。
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>运算符的比较</title>
</head>
<body>
<div style="margin-left: 40px">
<!--算术运算符-->
<p th:text="${age+20}"></p>
<p th:text="${10 % 3}"></p>
<!--关系运算符-->
<p th:if="${age > 10}">年龄大于10</p>
<p th:if="${age gt 10}">年龄大于10</p>
<!--相等判断-->
<p th:if="${name1 == null}">name1是null</p>
<p th:if="${name2 ne null}">name2不是null</p>
<!--三步运算符-->
<p th:text="${isLogin == true ? '用户已登录' : '用户未登录'}"></p>
<!--三元运算符的嵌套-->
<p th:text="${isLogin == true ? (age gt 10 ? '用户已成年' : '用户未成年') : '用户未登录'}"></p>
</div>
</body>
</html>
执行结果:
7. Thymeleaf内置对象
模板引擎提供了一组内置的对象,这些内置的对象可以直接在模板中使用,这些对象由#
号开始引用,我们比较常用的内置对象:
①#request 表示 HttpServletRequest ;
②#session 表示 HttpSession 对象;
③session 本质上是一个Map集合,是#session的简单表示方式,用来获取session中指定的key的值;
#session.getAttribute("loginname") == session.loginname
controller存数据
@GetMapping("/baseObject")
public String baseObject(Model model, HttpServletRequest request,
HttpSession session){
model.addAttribute("name","Jack");
request.setAttribute("requestData","request作用域中的对象");
session.setAttribute("sessionData","session作用域中的对象");
return "baseObject";
}
一:获取作用域中的数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p th:text="${name}"></p>
<p th:text="${#request.getAttribute('name')}"></p>
<!--#request,本质是一个HttpServletRequest-->
<p th:text="${#request.getAttribute('requestData')}"></p>
<!--#session,t,本质是一个HttpSession-->
<p th:text="${#session.getAttribute('sessionData')}"></p>
<!--session,本质是一个Map-->
<p th:text="${session.sessionData}"></p>
</div>
</body>
</html>
执行结果:
思考:剖析session对象;实际上session对象本质上是一个Map,存入的数据以key和value的形式存储,所以session.key的方式底层实际上就是调用map.get(key)的方式取出value数据
二:使用内置对象的方法
①getRequestURL(),获取完整的地址信息(不包含参数);
②getRequestURI(),获取地址信息(不包含IP地址、端口号、参数);
③getQueryString(),获取参数信息;
④getContextPath(),获取根路径;
⑤getServerName(),获取IP地址;
⑥getServerPort(),获取端口号;
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置对象</title>
</head>
<body>
<div style="margin-left: 400px">
<!--完整地址-->
<p th:text="${#request.getRequestURL()}"></p>
<!--相对的地址-->
<p th:text="${#request.getRequestURI()}"></p>
<!--参数-->
<p th:text="${#request.getQueryString()}"></p>
<!--根路径-->
<p th:text="${#request.getContextPath()}"></p>
<!--IP地址-->
<p th:text="${#request.getServerName()}"></p>
<!--端口号-->
<p th:text="${#request.getServerPort()}"></p>
</div>
</body>
</html>
执行结果:
8. Thymeleaf内置工具类对象
①模板引擎提供的一组功能性内置对象,可以在模板中直接使用这些对象提供的功能方法;
②工作中常使用的数据类型,如集合,时间,数值,可以使用 Thymeleaf 的提供的功能性对 象来处理它们;
③内置功能对象前都需要加#号,内置工具类对象一般都以 s 结尾;
Cat类
package com.zl.vo;
public class Cat {
private String name;
public Cat() {
}
public Cat(String name) {
this.name = name;
}
@Override
public String toString() {
return "Cat{" +
"name='" + name + '\'' +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Dog类
package com.zl.vo;
public class Dog {
private String name;
public Dog() {
}
public Dog(String name) {
this.name = name;
}
@Override
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Zoo类
package com.zl.vo;
public class Zoo {
private Cat cat;
private Dog dog;
public Zoo() {
}
public Zoo(Cat cat, Dog dog) {
this.cat = cat;
this.dog = dog;
}
@Override
public String toString() {
return "Zoo{" +
"cat=" + cat +
", dog=" + dog +
'}';
}
public Cat getCat() {
return cat;
}
public void setCat(Cat cat) {
this.cat = cat;
}
public Dog getDog() {
return dog;
}
public void setDog(Dog dog) {
this.dog = dog;
}
}
controller存数据
package com.zl.controller;
import com.zl.pojo.Student;
import com.zl.vo.Cat;
import com.zl.vo.Dog;
import com.zl.vo.Zoo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.security.PublicKey;
import java.util.*;
@Controller
@RequestMapping("/thymeleaf")
public class ThymeleafExpressionController {
@GetMapping("/utilObject")
public String utilObject(Model model){
model.addAttribute("mydate",new Date());
model.addAttribute("number",32.636);
model.addAttribute("str","hello");
List<String> list = Arrays.asList("Jack","Rose","King");
model.addAttribute("list",list);
Cat cat = new Cat("小猫");
Dog dog = new Dog("二哈");
zoo.setCat(cat);
// zoo.setDog(dog);
model.addAttribute("zoo",zoo);
return "utilObject";
}
}
一:#dates---处理日期的
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置工具类对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p>日期工具类对象#dates</p>
<!--默认的格式化方式-->
<p th:text="${#dates.format(mydate)}"></p>
<!--自定义格式化方式-->
<p th:text="${#dates.format(mydate,'yyyy-MM-dd')}"></p>
<p th:text="${#dates.format(mydate,'yyyy-MM-dd HH:mm:sss')}"></p>
<!--只获取对应的年月日-->
<p th:text="${#dates.year(mydate)}"></p>
<p th:text="${#dates.month(mydate)}"></p> <!--数字-->
<p th:text="${#dates.monthName(mydate)}"></p><!--字符串-->
<p th:text="${#dates.day(mydate)}"></p>
<!--获取当前的时间-->
<p th:text="${#dates.createNow()}"></p>
</div>
</body>
</html>
执行结果:
二:#numbers---处理数字的
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置工具类对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p>数字工具类对象#dates</p>
<!--格式化,带有货币符号,还会四舍五入-->
<p th:text="${#numbers.formatCurrency(number)}"></p>
<!--保留整数多少位,保留小数多少位-->
<p th:text="${#numbers.formatDecimal(number,5,2)}"></p>
</div>
</body>
</html>
执行结果:
三:#strings---处理字符串的
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置工具类对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p>字符串工具类对象#strings</p>
<!--转大写-->
<p th:text="${#strings.toUpperCase(str)}"></p>
<!--某个字符串在当前字符串存在的下标-->
<p th:text="${#strings.indexOf(str,'ll')}"></p>
<!--截取串-->
<p th:text="${#strings.substring(str,1,3)}"></p>
<!--字符串的拼接-->
<p th:text="${#strings.concat(str,'world')}"></p>
<!--字符串的长度-->
<p th:text="${#strings.length(str)}"></p>
</div>
</body>
</html>
执行结果:
四:#lists---处理集合的
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置工具类对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p>集合工具类对象#lists</p>
<!--集合中含有几个数据-->
<p th:text="${#lists.size(list)}"></p>
<!--是否包含Rose字符串-->
<p th:if="${#lists.contains(list,'Rose')}">list集合包含Rose</p>
<!--集合是否为空-->
<p th:text="${#lists.isEmpty(list)}"></p>
</div>
</body>
</html>
执行结果:
五:null处理
正常取出数据
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>内置工具类对象</title>
</head>
<body>
<div style="margin-left: 400px">
<p>处理null</p>
<!--正常取出数据-->
<p th:text="${zoo.dog.name}"></p>
<p th:text="${zoo.cat.name}"></p>
</div>
</body>
</html>
执行结果:
思考:如果此时对于cat没有赋上值
此时会出现500错误(服务器内部错误)
实际上是出现了空引用,如何避免呢?
在引用的后面使用问号?,如果引用存在就可以向下取属性;如果当前引用为空,直接不显示结果!
<p th:text="${zoo?.cat?.name}"></p>
执行结果:
9.自定义模板(内容复用)
自定义模板是复用的行为;可以把一些内容,多次重复使用!
模板语法
对于一个自定义模板的使用,主要包括两部分:
定义模板
模板定义语法:th:fragment="模板自定义名称"
<div th:fragment="head">
<p>
大连
</p>
<p>
www.dlu.com
</p>
</div>
使用模板
引用模板语法: ~{templatename :: selector} 或者 templatename :: selector
①其中templatename: 文件名称,selector: 自定义模板名称;
②对于使用模板:有包含模板(th:include), 插入模板(th:insert);
插入模板和包含模板
注:对于插入模板可以理解为整体的插入,对于包含模板可以理解为整体的替换!
第一步:模板的定义
head.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div th:fragment="top">
<p>www.baidu.com</p>
<p>百度官网</p>
</div>
</body>
</html>
第二步:欢迎页面index.html
<a href="thymeleaf/customtpl">自定义模板</a><br>
第三步:controller跳转到customtpl页面
@GetMapping("/customtpl")
public String customtpl(){
return "customtpl";
}
第四步:customtpl页面使用插入模板和包含模板
①插入模板
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div style="margin-left: 400px">
<h1>插入模板insert</h1>
<!--第一种方式-->
<div th:insert="~{head :: top}"></div>
<hr>
<!--第二种方式-->
<div th:insert="head :: top"></div>
</div>
</body>
</html>
执行结果:成功插入原来html页面的内容
F12打开前端代码分析:嵌套进去
②包含模板
<h1>包含模板include</h1>
<!--第一种方式-->
<div th:include="~{head :: top}"></div>
<hr style="color: red">
<!--第二种方式-->
<div th:include="head :: top"></div>
F12打开前端代码分析:完全替换
整个html页面作为模板插入
footer.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
footer.html
@www.baidu.com
</div>
</body>
</html>
此时把整个footer.html页面插入到 customtpl页面
注:前面主要是将某一个代码片段引入到其它页面,这里讲解的是将整个html页面引入到其它页面。
语法格式:
①<th:include/insert="文件名 :: 文件格式后缀名">
②<th:include/insert="文件名">省略后缀名
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div style="margin-left: 400px">
<h1>引入整个footer.html到此页面</h1>
<!--第一种方式-->
<p th:insert="footer :: html"></p>
<!--第二种方式-->
<p th:insert="footer"></p>
</div>
</body>
</html>
成功引入:
思考:以上是同级目录下的整个html页面的引入,如果是其它目录呢?
在templates目录下有一个common目录,common目录下有一个left.html
left.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>我是一个标签</h1>
</body>
</html>
引入其它目录下的页面到此页面
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div style="margin-left: 400px">
<h1>引入其它目录下的页面到此页面</h1>
<!--第一种方式-->
<p th:insert="common/left :: html"></p>
<!--第二种方式-->
<p th:insert="common/left"></p>
</div>
</body>
</html>
执行结果:成功引入
图书推荐:《巧用ChatGPT轻松玩转新媒体运营》
本次送书 2本!
活动时间:截止到 2023-10-12 00:00:00。抽奖方式:利用程序进行抽奖。
参与方式:关注博主(只限粉丝福利哦)、点赞、收藏,评论区随机抽取,最多三条评论!
关键点
★超实用 通过80多个实战案例和操作技巧,使读者能够快速上手并灵活运用ChatGPT技术及提高运营能力。
★巨全面 涵盖10多个新媒体领域,文案写作+图片制作+社交媒体运营+爆款视频文案+私域推广+广告策划+电商平台高效运营等。
★真好懂 以通俗易懂的语言解释ChatGPT的原理及应用,轻松提高新媒体运营能力。
★高回报 学习本书,全面提升运营能力,大大提高工作效率,促进职业发展,实现自我价值。
内容简介
本书从ChatGPT的基础知识讲起,针对运营工作中的各种痛点,结合实战案例,如文案写作、图片制作、社交媒体运营、爆款视频文案、私域推广、广告策划、电商平台高效运营等,手把手教你使用ChatGPT进行智能化工作。此外,还介绍了通过ChatGPT配合Midjourney、D-ID等AI软件的使用,进一步帮助提高运营工作的效率。
本书内容通俗易懂,案例丰富,实用性较强,特别适合想要掌握ChatGPT对话能力的读者和各行各业的运营人员,如互联网运营人员、自媒体运营人员、广告营销人员、电商运营人员等。 另外,本书也适合作为相关培训机构的教材使用。
当当网链接:当当图书
京东的链接:京东安全
更多推荐
所有评论(0)